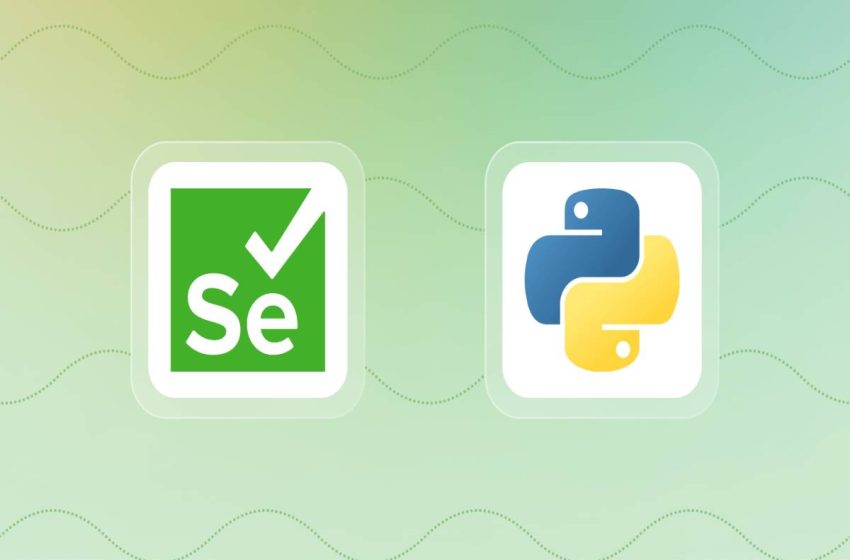
Selenium Python: A Step-by-Step Guide for Web Test Automation
Selenium Python
Web test automation has long been one of the cornerstones of efficient and effective software development. Automated web application testing allows teams to deliver consistent quality, saves much time on repetitive testing activities, and catches bugs earlier in the development cycle. Amongst the tools available for web test automation, Selenium Python represents one of the most popular and widely used frameworks. Selenium Python becomes a powerhouse and much more accessible when combined with the convenience of having a language principally known for its simplicity and readability: Python. This combination makes writing, maintaining, and scaling automated tests much easier, giving developers the flexibility to create robust and efficient test scripts for web applications.
This guide provides a walkthrough on Selenium’s basics using Python, as well as the major features surrounding the steps of setting up this powerful combination for web test automation.
Table of Contents
What is Selenium?
It allows testers to test web applications versus multiple browsers. Selenium encourages people to write test scripts similar in nature to how actual humans go around doing things: clicking the button, filling out forms, getting to pages, etc. Support of Chrome, Firefox, Safari, and Edge, places selenium as a pretty good cross-browser tester. Selenium can also be used not only with web applications but also with a number of programming languages – Python, Java, C#, and many more, making testing strategies flexible.
Why Use Python with Selenium?
Python is perhaps the most used programming language that is easily readable, and widely used nowadays. For multiple reasons, using Python along with Selenium is effective:
- Easy Learning: As part of its easy learning, the syntax for Python is easy and straightforward hence suitable for beginners to learn from it as well as to allow rapid development.
- Strong Ecosystem: There are rich libraries and frameworks that complement Selenium, including pytest to organize tests and requests for handling HTTP requests.
- Huge Community: This also means a treasure trove of available resources, tutorials, and forums for support.
These factors make Python an ideal language for automation testing, especially for those new to coding or automation.
Setting Up Your Environment
Before diving into automation testing with Selenium and Python, you need to set up your testing environment. This setup process involves a few essential steps:
- Install Python
To begin, you need to have Python installed on your machine. Here’s how you can do it:
Download: Visit the official Python website and download the latest version.
Install: Run the installer and ensure to check the box that says “Add Python to PATH.” This step is crucial for accessing Python from the command line.
- Install Selenium
The next step after installing Python is to install the Selenium library. This can be accomplished with Python’s package manager, pip. Open the command line interface (CLI) and execute the following command:
pip install selenium
This command will download and install the latest version of Selenium.
- Set Up WebDriver
Selenium requires a WebDriver for the browser you intend to test. A WebDriver serves as an intermediary between your Selenium scripts and the browser. Here’s how to do this:
Choose a WebDriver: Depending on the browser you want to automate, download the corresponding WebDriver:
- For Chrome, download ChromeDriver.
- For Firefox, download GeckoDriver.
Add WebDriver to PATH: After downloading, extract the WebDriver and place it in a directory. Ensure this directory is included in your system’s PATH variable so that Selenium can find the WebDriver.
Writing Your First Test
Now that you know all this, let’s write your first automated test using Selenium with Python. This process involves a few straightforward steps:
- Initialize the WebDriver
To begin, you need to initialize the WebDriver for your preferred browser. This command opens a browser session.
- Open a Web Page
Next, direct the browser to navigate to a specific URL, such as the homepage of your web application.
- Validate the Page Title
To ensure that the page has loaded correctly, you can print or verify the title of the webpage. This step confirms that your script successfully reached the desired location.
Using LambdaTest for automation testing
If you wish to run your tests on many browsers or platforms, consider including LambdaTest in your testing approach. LambdaTest is a cloud-based tool that allows you to automate Selenium testing across 3000 browsers and operating systems without having to handle the infrastructure. You can configure your WebDriver to run your tests on LambdaTest by specifying the required capabilities, allowing you to easily evaluate your application’s functionality across several environments.
- Close the Browser
Finally, after validating the page title, you should close the browser session to complete your test.
Locating Elements
Locating an element on the web page is one of the most crucial features of Selenium. It provides various kinds of methods for locating elements:
- By ID: This method finds an element based on its unique ID attribute.
- By Name: This method locates elements by their name attribute.
- By XPath: This flexible method allows you to navigate through elements and attributes in the XML document.
- By CSS Selector: This method uses CSS selectors to find elements based on their styles.
- By Class Name: This method locates elements that have a specific class attribute.
Understanding how to effectively locate elements is fundamental to interacting with them during testing.
Interacting with Elements
Once you can locate elements on a webpage, you can perform various interactions. Here are some common interactions:
- Entering Text
You can simulate user input by entering text into form fields. This action is crucial for testing user registration or login processes.
- Clicking Buttons
You can simulate clicking buttons or links to navigate through your application or submit forms. This interaction allows you to test the application’s flow.
- Retrieving Text
Selenium allows you to retrieve text from specific elements, which is essential for verifying expected outcomes. You can check if the correct message appears after an action, such as submitting a form.
Assertions in Selenium
Assertions are important in automated testing because they assist confirm that the expected outcomes match the actual results. Using assertions successfully ensures that your web application performs as expected. Assertions can be used to check criteria like whether the correct page title is displayed or if a specific text is included on the page.
Common Assertion Types
- Equality Assertions: Check if two values are equal.
- Presence Assertions: Verify that a particular element is present on the page.
- Visibility Assertions: Ensure that an element is visible to the user.
Implementing assertions in your tests increases confidence in the reliability of your web application.
Handling Alerts and Pop-ups
Web applications often use alerts and pop-ups to convey information or prompt user actions. Selenium provides functionalities to handle these alerts effectively. Here’s how:
Switching to an Alert
When an alert appears, you can switch the WebDriver’s focus to the alert to interact with it.
Accepting or Dismissing Alerts
You can choose to accept the alert (similar to clicking “OK”) or dismiss it (like clicking “Cancel”). This functionality allows you to automate tests that involve user confirmations.
Using Waits in Selenium
Waits are crucial in automation testing to synchronize the execution of your test scripts with the behavior of the web application. There are two main types of waits:
- Implicit Wait: An implicit wait determines the default waiting time for the entire WebDriver instance. This means that while attempting to find items, Selenium will wait for a set amount of time before throwing an exception.
- Explicit Wait: Explicit waits are more flexible, allowing you to wait for specified requirements to be met before advancing. This strategy is very beneficial for dealing with elements that take long to appear or become intractable.
By implementing waits effectively, you can reduce the chances of encountering errors due to timing issues.
Running Tests in Headless Mode
Headless mode lets you run tests without the graphical user interface. This makes your tests run much faster than normal, which makes it well-suited for CI/CD configurations. Headless testing is very useful in cases where tests need to be run on servers or cloud-based systems without any GUI.
Integrating with CI/CD
Integrating Selenium tests within the CI/CD pipeline would improve your testing practice in that it ensures that the test is run automatically every time there occurs a change in the code. Here is how one could set up this integration:
- Choosing a CI/CD Tool
Choice can be made from some of the CI/CD tools such as Jenkins, Travis CI, or even GitHub Actions to set up the automated testing environment.
- Setting Up Your Pipeline
Once you’ve chosen a CI/CD tool, you’ll need to configure it to run your test scripts. The typical process includes:
- Creating a Test Script: Write your Selenium tests as Python scripts.
- Executing Tests on Push: Configure the pipeline to trigger tests whenever code is pushed to the repository.
- Configuring the CI/CD Tool: Set up the CI/CD tool to execute your test scripts automatically.
- Configuring the CI/CD Tool: Set up the CI/CD tool to execute your test scripts automatically.
- Executing Tests on Push: Configure the pipeline to trigger tests whenever code is pushed to the repository.
This integration ensures that every code change is validated by running automated tests, allowing for quick feedback and continuous improvement.
Best Practices for Selenium Python Automation Testing
The best practices to optimize the effectiveness of your Selenium automation testing are:
- Use the Page Object Model (POM)
The POM design pattern improves the maintainability and readability of your test scripts. You can develop a more structured and manageable test suite by separating your code into different classes for each page of your application.
- Keep Tests Independent
Ensure that each test case is independent and does not rely on the outcome of another. This practice allows for easier debugging and parallel execution of tests.
- Implement Logging and Reporting
Integrate logging and reporting mechanisms into your tests to track execution and results. This approach will help you identify failures and analyze test performance over time.
- Regularly Review and Refactor Tests
As your application evolves, regularly review and refactor your test scripts to ensure they remain relevant and effective.
- Explore Advanced Features
Selenium provides advanced features such as handling frames, multiple windows, and working with dynamic content. Familiarize yourself with these capabilities to enhance your testing proficiency.
Conclusion
Selenium Python is a very effective mix for web test automation. Selenium’s simple syntax and comprehensive support for numerous web browsers enable you to automate repetitive testing processes, ensuring the quality and dependability of web applications. By following the methods indicated in this book, you may configure Selenium with Python and begin creating and executing your own test scripts. Remember to follow recommended practices to keep your test automation framework robust and easy to read.